How to architect a React application as a beginner?
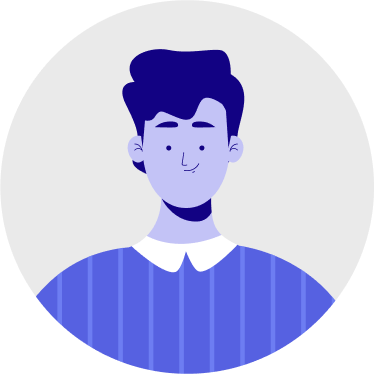
As a react developer, you may have created web pages or small web application using basic concepts such as creating the reusable components and handle component rendering using react-router-dom library and state. Yet, we should learn advance concepts and pre defined architecture model that can help to us build more complex and scalable applications. In this article we will learn some of those advanced concepts step by step and I will also share some blogs regarding it.
Pre-defined patterns or architectures in react
When it comes to structuring a React application, there are a few different Pre-defined models or architectures that provide a structure for organizing and managing the code within the application . Examples of such models include the Model-View-Controller (MVC) architecture and the Flux architecture. By following these pre-defined models, developers can create more maintainable, scalable, and efficient applications as they provide clear guidelines and responsibilities for each component within the application.
1. MVC (Model-View-Controller):-
MVC separates an application into three main components: Model, View, and Controller. The Model represents the data and logic of the application, the View displays the data to the user, and the Controller handles user input and updates the Model and View accordingly.
In a React application, you can implement MVC by creating separate folders for Models, Views, and Controllers. For example, you could have a models folder that contains your data models, a views folder that contains your React components, and a controllers folder that contains event handlers and other business logic.
// Example of a Model file
class User {
constructor(name, email) {
this.name = name;
this.email = email;
}
getFullName() {
return this.name;
}
}
// Example of a View file
import React from 'react';
import User from '../models/User';
function UserProfile(props) {
const user = new User(props.name, props.email);
return (
<div>
<h1>{ user.getFullName() }</h1>
<p>{ user.email }</p>
</div>
);
}
export default UserProfile;
// Example of a Controller file
function handleFormSubmit(event) {
event.preventDefault();
// perform form validation
// update user data
}
2. Flux developed by Facebook :
Flux is the application architecture that Facebook uses for building client-side web applications. . It emphasizes a unidirectional flow of data, where data flows from the Actions to the Dispatcher to the Stores and finally to the Views.
Actions are simple JavaScript objects that represent events in the system. They are dispatched to the Dispatcher, which is responsible for distributing the actions to the Stores. Stores are where the application state is stored, and they respond to Actions by updating their state and emitting a change event. Views subscribe to the Stores and update themselves accordingly.
// Example of an Action file
const UserActions = {
updateUser: (userData) => {
return {
type: 'UPDATE_USER',
payload: userData,
};
},
};
// Example of a Dispatcher file
import { Dispatcher } from 'flux';
const AppDispatcher = new Dispatcher();
AppDispatcher.register((action) => {
switch (action.type) {
case 'UPDATE_USER':
UserStore.updateUser(action.payload);
UserStore.emitChange();
break;
// handle other actions
}
});
// Example of a Store file
import { EventEmitter } from 'events';
const CHANGE_EVENT = 'change';
class UserStore extends EventEmitter {
constructor() {
super();
this.user = {};
}
updateUser(userData) {
this.user = { ...this.user, ...userData };
}
getUser() {
return this.user;
}
emitChange() {
this.emit(CHANGE_EVENT);
}
addChangeListener(callback) {
this.on(CHANGE_EVENT, callback); } }
A common folder structure for a React application ,regardless of pre-defined patterns.
If you do not like to follow a pre-defined pattern ,that's not a problem. However, it's important to use a common folder structure while developing a React application , because it helps to organize the code in a clear and efficient manner. Below is a general description of the most common folder structure:
src/
components/
common/
pages/
config/
hooks/
context/
services/
models/
store/
styles/
utils/
App.js
index.js
Conclusion
In conclusion ,designing a react application can be a difficult task because of its flexibility that gives us freedom to follow any kind of structure but as a developer gains more experience and work on different kinds of critical projects, they learn various different new techniques and patterns for creating a maintainable and scalable application. They always take new problems as an opportunity to learn and grow themselves. So, I advise all novice developers out there, keep on coding and never stop learning!